Wildfrost Mod
About the Game
Wildfrost is a tactical roguelike deckbuilding game, in which players journey across a frozen tundra, collecting cards strong enough to banish the eternal winter! Perfect your card battling and deck-building skills, and set out on a quest to bring an end to The Wildfrost!
This mod currently adds over 90 more units, items, and charms. There are more features planned as it's still in development, such as custom enemies and bosses, new regions and visuals, and much more!
Developed from November 2024 and is currently ongoing.
Role in Development
- Artist: DandyDingo
- Programmer: David Liu
- Designers: David Liu, DandyDingo
Wildfrost is developed in the Unity game engine, but mod support for the game relies on creating a DLL. Custom cards are created using builders that are part of the Wildfrost modding API, whereas Harmony is used for patching and injections into the source code. So far, I have only built upon the source code just using the Wildfrost modding API.
The mod started as a side passion project with a friend. We both really liked the game Wildfrost and wanted to keep playing more of it after completing the game, so we decided to create more of our own content and share our love of the game with others.
The artist I was working with isn't very experienced in game development, so I took the lead in getting the project off the ground. DandyDingo began to create ideas and keep them in a shared Google Doc while I began learning about the Wildfrost modding API and started creating a few basic cards. With my extensive knowledge of C# and Unity, I was able to quickly learn and understand the framework within just a couple days. Most of the effects for units are created using abstract classes with the use of enumerators and event callbacks. Within a week, I already had created ten custom cards with their own custom effects.
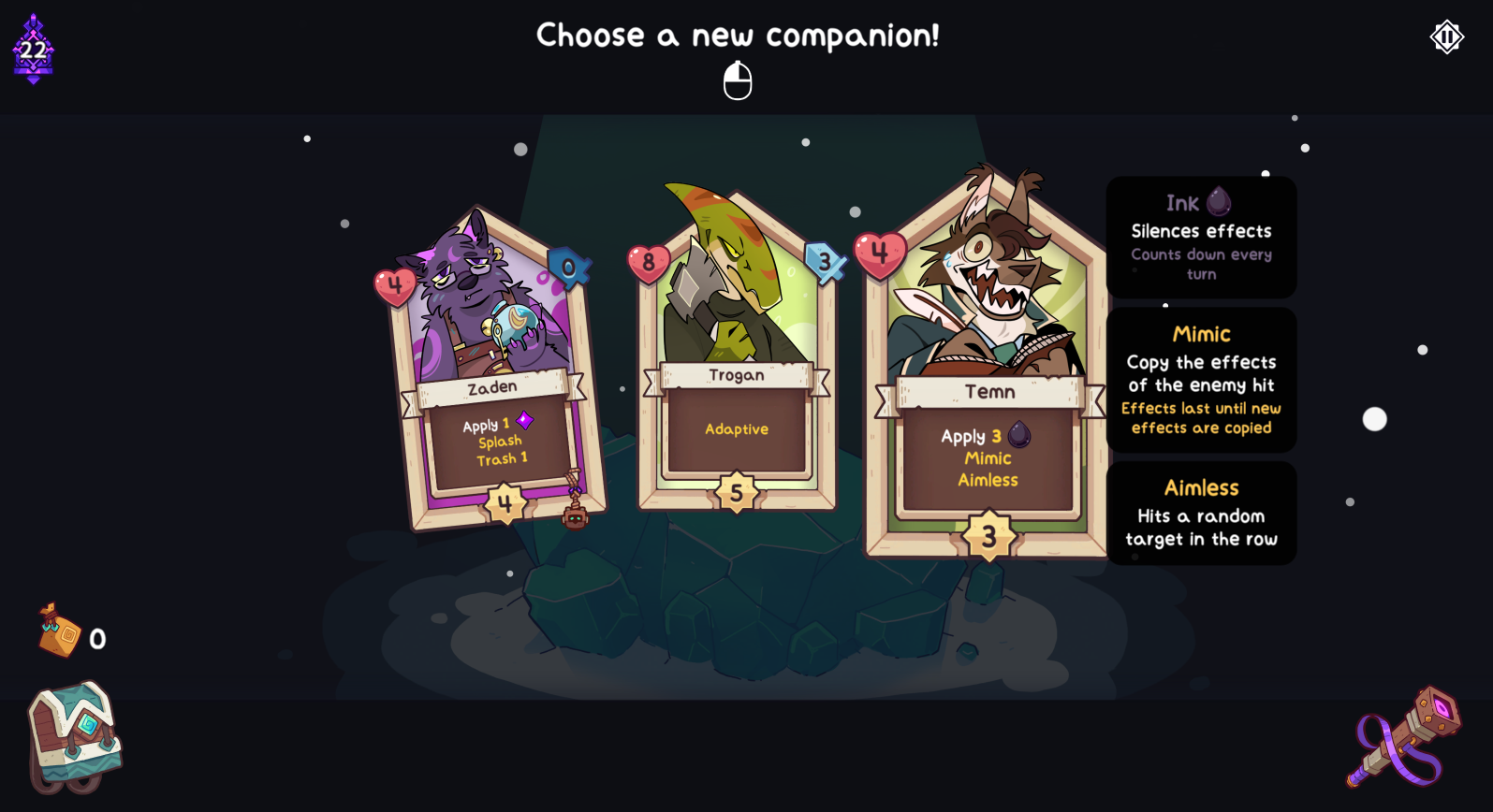
DandyDingo began working on the art for the custom cards in their free time. We were able to establish a good workflow between us, where they give me new batches of art following a specific naming convention, and I was able to easily place the files into the mod with minimal changes. This is the process we've kept up to this day.
Finding balance with the custom cards has proven to be a challenge. Although we both have good intuition from playing this game for hundreds of hours, the game has a little room between significant levels of power. Even changing the stats of a unit by one has made significant impacts on its capability in battles. DandyDingo and I also have very different playstyles, which is both good and bad because we are able to make diverse ideas together, but we also have different opinions on how powerful a card can be. We resolve most of our balance tweaks through continuous playtesting and reflecting back on recorded data.
We plan to continue developing this mod further with much more added content, since we both have still been having a lot of fun with this project. Once we reach our finalized product, we want to upload the mod to the Steam workshop and advertise it within small communities. Depending on our work-life balance at that point, we may continue to make more updates to the mod in the future. I'm very excited with the direction this passion project is going and can't wait to show more!
C# Examples
BaseMod.csTargetModeAdjacent.csTargetModeLowestHealthInRow.csStatusEffectInstantSwapPermanent.cs#region Function Overrides public override void Load() { Instance = this; // Save Data OriginalProfile = SaveSystem.GetProfile(); SwitchToSaveProfile("Dingofrost", copyFiles: true); if (!loaded) { CreateModAssets(); } base.Load(); CreateModBattles(); CustomModifications(); //needed for custom icons FloatingText ftext = GameObject.FindObjectOfType(true); ftext.textAsset.spriteAsset.fallbackSpriteAssets.Add(assetSprites); // Custom Tribe Fix Events.OnEntityCreated += FixImage; // Tribes GameMode gameMode = TryGet ("GameModeNormal"); gameMode.classes = gameMode.classes.Append(TryGet ("Wanderers")).ToArray(); // Save mod loaded data SwitchToSaveProfile(OriginalProfile); UpdateSave(); SwitchToSaveProfile("Dingofrost"); UpdateSave(); } public override void Unload() { base.Unload(); // Custom Tribe Fix Events.OnEntityCreated -= FixImage; GameMode gameMode = TryGet ("GameModeNormal"); gameMode.classes = RemoveNulls(gameMode.classes); //Without this, a non-restarted game would crash on tribe selection UnloadFromClasses(); //This tutorial doesn't need it, but it doesn't hurt to clean the pools // Save Data SwitchToSaveProfile(OriginalProfile); } public override List AddAssets () { return assets.OfType ().ToList(); } #endregion private void CreateModAssets() { // Needed to get sprites in text boxes assetSprites = CreateSpriteAsset("assetSprites", directoryWithPNGs: this.ImagePath("Sprites"), textures: new Texture2D[] { }, sprites: new Sprite[] { }); CreateModConstraints(); CreateModCards(); CreateModIcons(); } private void CreateModConstraints() { targetConstraints = new List (); #region Has Attack Effect TargetConstraintHasAttackEffect notInstantSummonCopy = ScriptableObject.CreateInstance (); notInstantSummonCopy.name = "Does Not Have Instant Summon Copy"; notInstantSummonCopy.effect = TryGet ("Instant Summon Copy"); notInstantSummonCopy.not = true; TargetConstraintHasAttackEffect notInstantSummonCopyOfEnemy = ScriptableObject.CreateInstance (); notInstantSummonCopyOfEnemy.name = "Does Not Have Instant Summon Copy Of Enemy"; notInstantSummonCopyOfEnemy.effect = TryGet ("Instant Summon Copy On Other Side With X Health"); notInstantSummonCopyOfEnemy.not = true; TargetConstraintHasAttackEffect notSacrificeAlly = ScriptableObject.CreateInstance (); notSacrificeAlly.name = "Does Not Have Sacrifice Ally"; notSacrificeAlly.effect = TryGet ("Sacrifice Ally"); notSacrificeAlly.not = true; #endregion #region Has Status TargetConstraintHasStatus hasConsume = ScriptableObject.CreateInstance (); hasConsume.name = "Has Consume"; hasConsume.status = TryGet ("Destroy After Use"); TargetConstraintHasStatus notHitAllEnemies = ScriptableObject.CreateInstance (); notHitAllEnemies.name = "Does Not Have Hit All Enemies"; notHitAllEnemies.status = TryGet ("Hit All Enemies"); notHitAllEnemies.not = true; #endregion #region Has Trait TargetConstraintHasTrait notAimless = ScriptableObject.CreateInstance (); notAimless.name = "Does Not Have Aimless"; notAimless.trait = TryGet ("Aimless"); notAimless.not = true; TargetConstraintHasTrait notBarrage = ScriptableObject.CreateInstance (); notBarrage.name = "Does Not Have Barrage"; notBarrage.trait = TryGet ("Barrage"); notBarrage.not = true; TargetConstraintHasTrait notLongshot = ScriptableObject.CreateInstance (); notLongshot.name = "Does Not Have Longshot"; notLongshot.trait = TryGet ("Longshot"); notLongshot.not = true; #endregion #region Play On Slot TargetConstraintPlayOnSlot playsOnBoard = ScriptableObject.CreateInstance (); playsOnBoard.name = "Plays On Board"; playsOnBoard.board = true; TargetConstraintPlayOnSlot notSlot = ScriptableObject.CreateInstance (); notSlot.name = "Does Not Play On Slot"; notSlot.slot = true; notSlot.not = true; #endregion #region Max Counter More Than TargetConstraintMaxCounterMoreThan counterMoreThan0 = ScriptableObject.CreateInstance (); counterMoreThan0.name = "Max Counter More Than 0"; #endregion #region Is Card Type TargetConstraintIsCardType notBoss = ScriptableObject.CreateInstance (); notBoss.name = "Is Not Boss"; notBoss.allowedTypes = new CardType[] { TryGet ("Boss") }; notBoss.not = true; TargetConstraintIsCardType notMiniboss = ScriptableObject.CreateInstance (); notMiniboss.name = "Is Not Miniboss"; notMiniboss.allowedTypes = new CardType[] { TryGet ("Miniboss") }; notMiniboss.not = true; TargetConstraintIsCardType notSmallBoss = ScriptableObject.CreateInstance (); notSmallBoss.name = "Is Not Small Boss"; notSmallBoss.allowedTypes = new CardType[] { TryGet ("BossSmall") }; notSmallBoss.not = true; #endregion #region Miscellaneous TargetConstraintDoesAttack doesAttack = ScriptableObject.CreateInstance (); doesAttack.name = "Does Attack"; TargetConstraintDoesDamage doesDamage = ScriptableObject.CreateInstance (); doesDamage.name = "Does Damage"; TargetConstraintHasHealth hasHealth = ScriptableObject.CreateInstance (); hasHealth.name = "Has Health"; TargetConstraintHasReaction hasReaction = ScriptableObject.CreateInstance (); hasReaction.name = "Has Reaction"; TargetConstraintIsUnit isUnit = ScriptableObject.CreateInstance (); isUnit.name = "Is Unit"; TargetConstraintIsItem isItem = ScriptableObject.CreateInstance (); isItem.name = "Is Item"; TargetConstraintNeedsTarget needsTarget = ScriptableObject.CreateInstance (); needsTarget.name = "Needs Target"; #endregion #region Or TargetConstraintOr doesTrigger = ScriptableObject.CreateInstance (); doesTrigger.name = "Does Trigger"; doesTrigger.constraints = new TargetConstraint[] { hasReaction, counterMoreThan0, isItem }; TargetConstraintOr hasHealthOrAttack = ScriptableObject.CreateInstance (); hasHealthOrAttack.name = "Has Health Or Attack"; hasHealthOrAttack.constraints = new TargetConstraint[] { hasHealth, doesDamage }; TargetConstraintOr isUnitOrNeedsTarget = ScriptableObject.CreateInstance (); isUnitOrNeedsTarget.name = "Is Unit Or Needs Target"; isUnitOrNeedsTarget.constraints = new TargetConstraint[] { isUnit, needsTarget }; #endregion targetConstraints.AddRange(new TargetConstraint[] { // Has Attack Effect notInstantSummonCopy, notInstantSummonCopyOfEnemy, notSacrificeAlly, // Has Status hasConsume, notHitAllEnemies, // Has Trait notAimless, notBarrage, notLongshot, // Play On Slot playsOnBoard, notSlot, // Max Counter More Than counterMoreThan0, // Is Card Type notBoss, notMiniboss, notSmallBoss, // Miscellaneous doesAttack, doesDamage, hasHealth, hasReaction, isUnit, isItem, needsTarget, // Or doesTrigger, hasHealthOrAttack, isUnitOrNeedsTarget }); } private void CreateModCards() { #region Tribes assets.Add( TribeCopy("Clunk", "Wanderers") .WithFlag("Images/Tribes/Wanderer_Tribe_Flag.png") .WithSelectSfxEvent(FMODUnity.RuntimeManager.PathToEventReference("event:/sfx/status/bom_hit")) .SubscribeToAfterAllBuildEvent( (data) => { // Game Object GameObject gameObject = data.characterPrefab.gameObject.InstantiateKeepName(); //Copy the previous prefab UnityEngine.Object.DontDestroyOnLoad(gameObject); //GameObject may be destroyed if their scene is unloaded. This ensures that will never happen to our gameObject gameObject.name = "Player (Wanderers)"; //For comparison, the clunkmaster character is named "Player (Clunk)" data.characterPrefab = gameObject.GetComponent (); //Set the characterPrefab to our new prefab data.id = "Wanderers"; //Used to track win/loss statistics for the tribe. Not displayed anywhere though :/ // Leaders data.leaders = new CardData[] { TryGet ("tom"), TryGet ("dingo"), TryGet ("dandy") }; // Starting Deck Inventory inventory = ScriptableObject.CreateInstance (); CardDataList startingDeck = new CardDataList(); startingDeck.Add(TryGet ("hatchet")); startingDeck.Add(TryGet ("hatchet")); startingDeck.Add(TryGet ("hatchet")); startingDeck.Add(TryGet ("hatchet")); startingDeck.Add(TryGet ("frozenfire")); startingDeck.Add(TryGet ("rations")); startingDeck.Add(TryGet ("playingcards")); startingDeck.Add(TryGet ("sewingkit")); inventory.deck = startingDeck; data.startingInventory = inventory; // Reward Pools RewardPool unitPool = CreateRewardPool("WanderersUnitPool", "Units", new DataFile[] { TryGet ("Elysio"), TryGet ("fiend"), TryGet ("temn"), TryGet ("captainzoryn"), TryGet ("pixel"), TryGet ("zaden"), TryGet ("salem"), TryGet ("razz"), TryGet ("harriot"), TryGet ("peppermint"), TryGet ("covet"), TryGet ("trogan"), TryGet ("buttercup"), TryGet ("jasper"), TryGet ("stasis"), TryGet ("Priscylla"), TryGet ("Felice"), TryGet ("Brewster"), TryGet ("Ether"), TryGet ("Steven"), TryGet ("Joseph"), TryGet ("Hugo"), TryGet ("Hullaballoo"), TryGet ("Oryx"), TryGet ("Atticus"), TryGet ("Kreedance"), TryGet ("Ren"), TryGet ("Snip"), TryGet ("Brew"), TryGet ("Barb") }); RewardPool itemPool = CreateRewardPool("WanderersItemPool", "Items", new DataFile[] { // Items TryGet ("Whiskey"), TryGet ("Smokes"), TryGet ("Painkillers"), TryGet ("ConfusingMap"), TryGet ("SoulCandy"), TryGet ("Book"), TryGet ("RageBrew"), TryGet ("MercifulDagger"), TryGet ("Cutlass"), TryGet ("WornDice"), TryGet ("Quill"), TryGet ("FoulSoup"), TryGet ("HuntingSpear"), TryGet ("ThermalTracker"), TryGet ("Pickaxe"), TryGet ("VolatileVial"), TryGet ("BarbedWire"), TryGet ("SunCookies"), TryGet ("ScytheShard"), TryGet ("PaidContract"), TryGet ("GoodSoup"), TryGet ("Bomb"), TryGet ("ReligiousText"), TryGet ("OtherworldlyFeather"), TryGet ("HauntedSword"), TryGet ("AssortedPeppers"), TryGet ("ForagingBasket"), TryGet ("SpoiledRations"), //TryGet ("TinySnowman"), TryGet ("MassiveSnowflake"), TryGet ("BottledDream"), TryGet ("TastyPage"), TryGet ("CursedScythe"), TryGet ("Cage"), TryGet ("BloodTrail"), TryGet ("FaintShadow"), TryGet ("SnakeOil"), TryGet ("SoothingTea"), // Clunkers TryGet ("Firewood"), TryGet ("Radio"), TryGet ("FrostedTree"), TryGet ("NooseTrap"), TryGet ("Booty"), TryGet ("Effigy"), TryGet ("Bookshelf"), TryGet ("VultureTotem") }); RewardPool charmPool = CreateRewardPool("WanderersCharmPool", "Charms", new DataFile[] { TryGet ("CardUpgradeHunter"), TryGet ("CardUpgradeScavenger"), TryGet ("CardUpgradeHex"), TryGet ("CardUpgradeSplash"), TryGet ("CardUpgradeAdaptive") }); data.rewardPools = new RewardPool[] { unitPool, itemPool, charmPool, Extensions.GetRewardPool("GeneralCharmPool"), Extensions.GetRewardPool("GeneralModifierPool") }; }) ); #endregion #region Leaders #region Tom assets.Add( new CardDataBuilder(this).CreateUnit("tom", "Tom") .SetSprites("Characters/WandererTribe_CardArt_Tom.png", "Backgrounds/WandererTribe_CardBG_Tom.png") .SetStats(7, 1, 4) .WithCardType("Leader") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Creation scripts data.createScripts = new CardScript[] { GiveUpgrade() }; // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Ally Is Targeted Add Attack Or Health", 1) }; }) ); #endregion #region Dandy assets.Add( new CardDataBuilder(this).CreateUnit("dandy", "Dandy") .SetSprites("Characters/WandererTribe_CardArt_Dandy.png", "Backgrounds/WandererTribe_CardBG_Dandy.png") .SetStats(8) .WithCardType("Leader") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Creation scripts data.createScripts = new CardScript[] { GiveUpgrade() }; // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Hit Restore Health And Reduce Counter To Allies", 1) }; }) ); #endregion #region Dingo assets.Add( new CardDataBuilder(this).CreateUnit("dingo", "Dingo") .SetSprites("Characters/WandererTribe_CardArt_Dingo.png", "Backgrounds/WandererTribe_CardBG_Dingo.png") .SetStats(6, 2, 3) .WithCardType("Leader") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Creation scripts data.createScripts = new CardScript[] { GiveUpgrade() }; // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("While Active Increase Effects To Allies & Enemies (Fixed)", 1), SStack("Hex", 2) }; }) ); #endregion #endregion #region Companions #region Fiend assets.Add( new CardDataBuilder(this).CreateUnit("fiend", "Fiend") .SetSprites("Characters/WandererTribe_CardArt_Fiend.png", "Backgrounds/WandererTribe_CardBG_Fiend.png") .SetStats(8, 2, 4) .WithCardType("Friendly") .SetStartWithEffect(SStack("When Hit Draw", 1), SStack("On Kill Draw", 1)) ); #endregion #region Temn assets.Add( new CardDataBuilder(this).CreateUnit("temn", "Temn") .SetSprites("Characters/WandererTribe_CardArt_Temn.png", "Backgrounds/WandererTribe_CardBG_Temn.png") .SetStats(4, null, 3) .WithCardType("Friendly") .SetAttackEffect(SStack("Null", 3)) .SetTraits(TStack("Aimless", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Copy Effects", 1) }; }) ); #endregion #region Captain Zoryn assets.Add( new CardDataBuilder(this).CreateUnit("captainzoryn", "Captain Zoryn") .SetSprites("Characters/WandererTribe_CardArt_CaptainZoryn.png", "Backgrounds/WandererTribe_CardBG_Zoryn.png") .SetStats(3, 3, 4) .WithCardType("Friendly") .SetTraits(TStack("Longshot", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Turn Drop Gold", 1), SStack("On Card Played Boost To Self", 1) }; }) ); #endregion #region Pixel assets.Add( new CardDataBuilder(this).CreateUnit("pixel", "Pixel") .SetSprites("Characters/WandererTribe_CardArt_Pixel.png", "Backgrounds/WandererTribe_CardBG_Pixel.png") .SetStats(3, 1, 3) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Card Is Consumed Add Attack To All Allies", 1) }; }) ); #endregion #region Zaden assets.Add( new CardDataBuilder(this).CreateUnit("zaden", "Zaden") .SetSprites("Characters/WandererTribe_CardArt_Berry.png", "Backgrounds/WandererTribe_CardBG_Berry.png") .SetStats(4, 0, 4) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Hex", 1) }; data.traits.Add(TStack("Splash", 1)); }) ); #endregion #region Salem assets.Add( new CardDataBuilder(this).CreateUnit("salem", "Salem") .SetSprites("Characters/WandererTribe_CardArt_Vultra.png", "Backgrounds/WandererTribe_CardBG_Vultra.png") .SetStats(10, 0, 5) .WithCardType("Friendly") .SetTraits(TStack("Smackback", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Bonus Damage Equal To Enemy Missing Health", 1) }; }) ); #endregion #region Razz assets.Add( new CardDataBuilder(this).CreateUnit("razz", "Razz") .SetSprites("Characters/WandererTribe_CardArt_Razz.png", "Backgrounds/WandererTribe_CardBG_Razz.png") .SetStats(6, 5, 4) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Reduce Counter Reduce Counter", 1) }; }) ); #endregion #region Harriot assets.Add( new CardDataBuilder(this).CreateUnit("harriot", "Harriot") .SetSprites("Characters/WandererTribe_CardArt_Harriot.png", "Backgrounds/WandererTribe_CardBG_Harriot.png") .SetStats(4, 1, 4) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Destroy Rightmost Card In Hand And Gain Attack", 1) }; }) ); #endregion #region Peppermint assets.Add( new CardDataBuilder(this).CreateUnit("peppermint", "Peppermint") .SetSprites("Characters/WandererTribe_CardArt_Peppermint.png", "Backgrounds/WandererTribe_CardBG_Peppermint.png") .SetStats(9) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Healed Apply Shroom To Random Enemy", 1) }; }) ); #endregion #region Covet assets.Add( new CardDataBuilder(this).CreateUnit("covet", "Covet") .SetSprites("Characters/WandererTribe_CardArt_Covet.png", "Backgrounds/WandererTribe_CardBG_Covet.png") .SetStats(1, 1, 2) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Deployed Swap Stats With Front Ally", 1) }; }) ); #endregion #region Trogan assets.Add( new CardDataBuilder(this).CreateUnit("trogan", "Trogan") .SetSprites("Characters/WandererTribe_CardArt_Trogan.png", "Backgrounds/WandererTribe_CardBG_Trogan.png") .SetStats(8, 3, 5) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Adaptive", 1) }; }) ); #endregion #region Buttercup assets.Add( new CardDataBuilder(this).CreateUnit("buttercup", "Buttercup") .SetSprites("Characters/WandererTribe_CardArt_Buttercup.png", "Backgrounds/WandererTribe_CardBG_Buttercup.png") .SetStats(4, 2, 3) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Trigger Against Allies In Row", 1) }; data.traits.Add(TStack("Scavenger", 2)); }) ); #endregion #region Jasper assets.Add( new CardDataBuilder(this).CreateUnit("jasper", "Jasper") .SetSprites("Characters/WandererTribe_CardArt_Jasper.png", "Backgrounds/WandererTribe_CardBG_Jasper.png") .SetStats(1) .WithCardType("Friendly") .SetTraits(TStack("Pigheaded", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Deployed Add Frenzy To Hand", 1) }; }) ); #endregion #region Stasis assets.Add( new CardDataBuilder(this).CreateUnit("stasis", "Stasis") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(5) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Deployed Summon Lion", 1) }; }) ); #endregion #region Priscylla assets.Add( new CardDataBuilder(this).CreateUnit("Priscylla", "Priscylla") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(4) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Trigger Against When Enemy Below Health X", 4) }; data.attackEffects = new CardData.StatusEffectStacks[] { SStack("Instant Kill", 1) }; }) ); #endregion #region Felice assets.Add( new CardDataBuilder(this).CreateUnit("Felice", "Felice") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(1, null, 2) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Sheep Coworker", 1), SStack("On Card Played Add Paperwork To Hand", 1) }; }) ); #endregion #region Brewster assets.Add( new CardDataBuilder(this).CreateUnit("Brewster", "Brewster") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(1, 0, 4) .WithCardType("Friendly") .SetTraits(TStack("Aimless", 1), TStack("Fragile", 1), TStack("Wild", 1), TStack("Pull", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("MultiHit", 2), SStack("Bloodlust", 1) }; }) ); #endregion #region Ether assets.Add( new CardDataBuilder(this).CreateUnit("Ether", "Ether") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(3, 0, 10) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Increase Counter To Target And Reduce Counter To Self", 1), SStack("On Turn Apply Attack To Self", 1), SStack("Trigger Against When Enemy Attacks", 1) }; }) ); #endregion #region Steven assets.Add( new CardDataBuilder(this).CreateUnit("Steven", "Steven") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(6, 2, 3) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("While Active In Full Board Gain Attack And Barrage", 3) }; }) ); #endregion #region Joseph assets.Add( new CardDataBuilder(this).CreateUnit("Joseph", "Joseph") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(3, 1, 4) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Sacrifice Adjacent Allies", 1) }; data.traits.Add(TStack("Scavenger", 3)); }) ); #endregion #region Hugo assets.Add( new CardDataBuilder(this).CreateUnit("Hugo", "Hugo") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(1, null, 4) .WithCardType("Friendly") .CanBeHit(false) // Intangible .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.attackEffects = new CardData.StatusEffectStacks[] { SStack("Overload", 1) }; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Intangible", 1), SStack("Nocturnal", 1), SStack("On Card Played Boost To Self", 1), SStack("Hit All Enemies", 1) }; }) ); #endregion #region Hullaballoo assets.Add( new CardDataBuilder(this).CreateUnit("Hullaballoo", "Hullaballoo") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(1, 3, 4) .WithCardType("Friendly") .SetStartWithEffect(SStack("Block", 1), SStack("On Card Played Apply Block To Self", 1)) ); #endregion #region Oryx assets.Add( new CardDataBuilder(this).CreateUnit("Oryx", "Oryx") .SetSprites("Characters/WandererTribe_CardArt_Stasis.png", "Backgrounds/WandererTribe_CardBG_Stasis.png") .SetStats(1, null, 2) .WithCardType("Friendly") .CanBeHit(false) // Intangible .SetTraits(TStack("Draw",1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Intangible", 1), SStack("Nocturnal", 1) }; }) ); #endregion #region Atticus assets.Add( new CardDataBuilder(this).CreateUnit("Atticus", "Atticus") .SetSprites("Characters/WandererTribe_CardArt_Trogan.png", "Backgrounds/WandererTribe_CardBG_Trogan.png") .SetStats(8, 0, 5) .WithCardType("Friendly") .SetAttackEffect(SStack("Overload", 3)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Adaptive", 1) }; }) ); #endregion #region Kreedance assets.Add( new CardDataBuilder(this).CreateUnit("Kreedance", "Kreedance") .SetSprites("Characters/WandererTribe_CardArt_Trogan.png", "Backgrounds/WandererTribe_CardBG_Trogan.png") .SetStats(12, 1) .WithCardType("Friendly") .SetTraits(TStack("Smackback", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Teeth", 3), SStack("Pre Card Played Apply Smackback To Target", 1) }; }) ); #endregion #region Ren assets.Add( new CardDataBuilder(this).CreateUnit("Ren", "Ren") .SetSprites("Characters/WandererTribe_CardArt_Trogan.png", "Backgrounds/WandererTribe_CardBG_Trogan.png") .SetStats(9, 2, 4) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Turn Heal Self And Allies", 2) }; }) ); #endregion #region Snip assets.Add( new CardDataBuilder(this).CreateUnit("Snip", "Snip") .SetSprites("Characters/WandererTribe_CardArt_Trogan.png", "Backgrounds/WandererTribe_CardBG_Trogan.png") .SetStats(2, 5, 2) .WithCardType("Friendly") .SetTraits(TStack("Pull", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Turn Randomize Stats (2-5)", 1), SStack("Adaptive", 1) }; }) ); #endregion #region Brew assets.Add( new CardDataBuilder(this).CreateUnit("Brew", "Brew") .SetSprites("Characters/WandererTribe_CardArt_Trogan.png", "Backgrounds/WandererTribe_CardBG_Trogan.png") .SetStats(11) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Hit Multiple 5 Instant Summon Big Fishy", 5) }; }) ); #endregion #region Barb assets.Add( new CardDataBuilder(this).CreateUnit("Barb", "Barb") .SetSprites("Characters/WandererTribe_CardArt_Trogan.png", "Backgrounds/WandererTribe_CardBG_Trogan.png") .SetStats(7, 2, 4) .WithCardType("Friendly") .SetAttackEffect(SStack("Null", 5)) ); #endregion #region Roséate assets.Add(new CardDataBuilder(this) .CreateUnit("Roseate", "Roseate") .SetSprites("Characters/WandererTribe_CardArt_Trogan.png", "Backgrounds/WandererTribe_CardBG_Trogan.png") .SetStats(2, 2, 3) .WithCardType("Friendly") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Instant Swap Effects", 1) }; }) ); #endregion #endregion #region Pets #region Rye assets.Add( new CardDataBuilder(this).CreateUnit("Rye", "Rye") .SetSprites("Characters/WandererTribe_CardArt_Fiend.png", "Backgrounds/WandererTribe_CardBG_Fiend.png") .SetStats(3, 3) .WithCardType("Friendly") .IsPet(String.Empty) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Discard Self", 1), SStack("Trigger Against Random Enemy When Drawn", 1), SStack("Destroy After Place", 1) }; }) ); #endregion #region Lionel assets.Add( new CardDataBuilder(this).CreateUnit("Lionel", "Lionel") .SetSprites("Characters/WandererTribe_CardArt_Fiend.png", "Backgrounds/WandererTribe_CardBG_Fiend.png") .SetStats(3, 2, 3) .WithCardType("Friendly") .IsPet(String.Empty) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Apply Bless To Random Ally", 1) }; }) ); #endregion #region Talon assets.Add( new CardDataBuilder(this).CreateUnit("Talon", "Talon") .SetSprites("Characters/WandererTribe_CardArt_Fiend.png", "Backgrounds/WandererTribe_CardBG_Fiend.png") .SetStats(2, 2, 4) .WithCardType("Friendly") .IsPet(String.Empty) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.traits.Add(TStack("Scavenger", 1)); }) ); #endregion #region Regus assets.Add( new CardDataBuilder(this).CreateUnit("Regus", "Regus") .SetSprites("Characters/WandererTribe_CardArt_Fiend.png", "Backgrounds/WandererTribe_CardBG_Fiend.png") .SetStats(6, 3, 3) .WithCardType("Friendly") .IsPet(String.Empty) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.traits.Add(TStack("Hunter", 1)); }) ); #endregion #endregion #region Summons #region Plush Monster assets.Add( new CardDataBuilder(this).CreateUnit("plushmonster", "Plush Monster") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .SetStats(1, null, 3) .WithCardType("Summoned") .SetTraits(TStack("Fragile", 1)) .SetStartWithEffect(SStack("On Card Played Trigger RandomAlly", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.startWithEffects[0].data.type = "On Card Played Trigger RandomAlly"; TargetConstraintHasStatusType constraint = ScriptableObject.CreateInstance (); constraint.statusType = "On Card Played Trigger RandomAlly"; constraint.not = true; TargetConstraintHasStatus hasSnow = ScriptableObject.CreateInstance (); hasSnow.status = TryGet ("Snow"); hasSnow.not = true; ((StatusEffectApplyX)data.startWithEffects[0].data).applyConstraints = ((StatusEffectApplyX)data.startWithEffects[0].data).applyConstraints.AddRangeToArray(new TargetConstraint[] { constraint, hasSnow, ScriptableObject.CreateInstance (), ScriptableObject.CreateInstance () }); }) ); #endregion #region Chuckles assets.Add( new CardDataBuilder(this).CreateUnit("chuckles", "Chuckles") .SetSprites("Characters/WandererTribe_CardArt_Chuckles.png", "Backgrounds/WandererTribe_CardBG_Chuckles.png") .SetStats(2, 2, 4) .WithCardType("Summoned") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.traits.Add(TStack("Scavenger", 2)); }) ); #endregion #region Steak assets.Add( new CardDataBuilder(this).CreateUnit("steak", "Steak") .SetSprites("Characters/WandererTribe_CardArt_Steak.png", "Backgrounds/WandererTribe_CardBG_Steak.png") .SetStats(8) .WithCardType("Summoned") .SetStartWithEffect(SStack("Teeth", 4)) ); #endregion #region Cleaver assets.Add( new CardDataBuilder(this).CreateUnit("cleaver", "Cleaver") .SetSprites("Characters/WandererTribe_CardArt_Cleaver.png", "Backgrounds/WandererTribe_CardBG_Cleaver.png") .SetStats(4, 4, 2) .WithCardType("Summoned") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.traits = new List () { TStack("Hunter", 1) }; }) ); #endregion #region Arsy Lion assets.Add( new CardDataBuilder(this).CreateUnit("arsyLion", "Arsy") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(1, null, 2) .WithCardType("Summoned") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Deployed Summon All Lions", 1), SStack("On Turn Escape To Self", 1) }; }) ); #endregion #region Innocent Monster assets.Add( new CardDataBuilder(this).CreateUnit("innocentMonster", "Innocent Monster") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(5, 5) .WithCardType("Summoned") .SetTraits(TStack("Smackback", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Destroyed Add Health To Enemies", 5) }; }) ); #endregion #region Frozen Corpse assets.Add( new CardDataBuilder(this).CreateUnit("frozencorpse", "Frozen Corpse") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(3, 1, 1) .WithCardType("Summoned") .SetAttackEffect(SStack("Snow", 2)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Block", 1), SStack("Stop Count Down While Self Has Block", 1) }; }) ); #endregion #region Sheep Coworker assets.Add( new CardDataBuilder(this).CreateUnit("SheepCoworker", "Sheep Coworker") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(1, null, 2) .WithCardType("Summoned") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Apply Health To Self", 1), SStack("On Card Played Add Paperwork To Hand", 1) }; }) ); #endregion #region Big Fishy assets.Add( new CardDataBuilder(this).CreateUnit("BigFishy", "Big Fishy") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(15, 15) .WithCardType("Summoned") .SetTraits(TStack("Barrage", 1), TStack("Smackback", 1)) ); #endregion #region Nightmare assets.Add( new CardDataBuilder(this).CreateUnit("Nightmare", "Nightmare") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(9, 1, 3) .WithCardType("Summoned") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Hex", 1), SStack("When Ally Is Sacrificed Boost Effects To Self", 1) }; }) ); #endregion #region Knowledge Gremlin assets.Add( new CardDataBuilder(this).CreateUnit("KnowledgeGremlin", "Knowledge Gremlin") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(3, 2) .WithCardType("Summoned") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Trigger When Card Drawn", 1) }; }) ); #endregion #region Savage Beast assets.Add(new CardDataBuilder(this) .CreateUnit("SavageBeast", "Savage Beast") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(2, 6, 3) .WithCardType("Summoned") ); #endregion #region Ravenous Creature assets.Add(new CardDataBuilder(this) .CreateUnit("RavenousCreature", "Ravenous Creature") .SetSprites("Characters/WandererTribe_CardArt_ArsyLion.png", "Backgrounds/WandererTribe_CardBG_ArsyLion.png") .SetStats(2, 2, 5) .WithCardType("Summoned") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Ally Summoned Sacrifice Ally And Instant Apply Current Health And Attack", 1) }; }) ); #endregion #region Protector assets.Add(new CardDataBuilder(this) .CreateUnit("TomShadow", "Protector") .SetSprites("Characters/WandererTribe_SummonArt_Protector.png", "Backgrounds/WandererTribe_SummonBG_Protector.png") .SetStats(1, 1, 4) .WithCardType("Summoned") .SetTraits(TStack("Fragile", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Ally Is Targeted Add Attack Or Health", 1) }; }) ); #endregion #region Caregiver assets.Add(new CardDataBuilder(this) .CreateUnit("DandyShadow", "Caregiver") .SetSprites("Characters/WandererTribe_SummonArt_Caregiver.png", "Backgrounds/WandererTribe_SummonBG_Caregiver.png") .SetStats(1) .WithCardType("Summoned") .SetTraits(TStack("Fragile", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("When Hit Restore Health And Reduce Counter To Allies", 1) }; }) ); #endregion #region Despoiler assets.Add(new CardDataBuilder(this) .CreateUnit("DingoShadow", "Despoiler") .SetSprites("Characters/WandererTribe_SummonArt_Despoiler.png", "Backgrounds/WandererTribe_SummonBG_Despoiler.png") .SetStats(1, 2, 3) .WithCardType("Summoned") .SetTraits(TStack("Fragile", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("While Active Increase Effects To Allies & Enemies (Fixed)", 1), SStack("Hex", 2) }; }) ); #endregion #endregion #region Clunkers #region Firewood assets.Add(new CardDataBuilder(this) .CreateUnit("Firewood", "Firewood", "TargetModeBasic", "") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .WithCardType("Clunker") .SetStats(null, null, 1) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.hasHealth = false; data.hasAttack = false; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Scrap", 1), SStack("On Turn Heal Allies In Row", 1) }; }) ); #endregion #region Radio assets.Add(new CardDataBuilder(this) .CreateUnit("Radio", "Radio", "TargetModeBasic", "") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .WithCardType("Clunker") .SetStats(null, null, 2) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.hasHealth = false; data.hasAttack = false; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Scrap", 1), SStack("On Turn Increase Attack To Allies In Row", 1) }; }) ); #endregion #region Frosted Tree assets.Add(new CardDataBuilder(this) .CreateUnit("FrostedTree", "Frosted Tree", "TargetModeBasic", "") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .WithCardType("Clunker") .SetTraits(TStack("Pigheaded", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.hasHealth = false; data.hasAttack = false; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Scrap", 1), SStack("While Active Increase Effects And Decrease Attack To Allies In The Row", 1) }; }) ); #endregion #region Noose Trap assets.Add(new CardDataBuilder(this) .CreateUnit("NooseTrap", "Noose Trap", "TargetModeBasic", "") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .WithCardType("Clunker") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.hasHealth = false; data.hasAttack = false; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Scrap", 1), SStack("When Destroyed Set Attacker Attack", 1) }; }) ); #endregion #region Pile O' Booty assets.Add(new CardDataBuilder(this) .CreateUnit("Booty", "Pile O' Booty", "TargetModeBasic", "") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .WithCardType("Clunker") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.hasHealth = false; data.hasAttack = false; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Scrap", 1), SStack("On Turn Drop Gold", 1), SStack("On Card Played Boost To Self", 1), SStack("Trigger When Enemy Is Killed", 1) }; }) ); #endregion #region Effigy assets.Add(new CardDataBuilder(this) .CreateUnit("Effigy", "Effigy", "TargetModeBasic", "") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .WithCardType("Clunker") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.hasHealth = false; data.hasAttack = false; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Scrap", 1), SStack("On Card Played Apply Bless To Allies", 1), SStack("Trigger When Ally Is Killed", 1) }; }) ); #endregion #region Bookshelf assets.Add(new CardDataBuilder(this) .CreateUnit("Bookshelf", "Bookshelf", "TargetModeBasic", "") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .WithCardType("Clunker") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.hasHealth = false; data.hasAttack = false; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Scrap", 1), SStack("On Card Played Draw Equal To Summoned Allies", 1), SStack("Trigger When Ally Summoned", 1) }; }) ); #endregion #region Vulture Totem assets.Add(new CardDataBuilder(this) .CreateUnit("VultureTotem", "Vulture Totem", "TargetModeBasic", "") .SetSprites("Items/WandererTribe_CardArt_PlushMonster.png", "Backgrounds/WandererTribe_ItemBG_PlushMonster.png") .WithCardType("Clunker") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.hasHealth = false; data.hasAttack = false; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Scrap", 1), SStack("On Card Played Instant Add Scavenger To Random Ally", 1), SStack("Trigger When Ally Is Sacrificed", 1) }; }) ); #endregion #endregion #region Enemies #region Dingo assets.Add( new CardDataBuilder(this).CreateUnit("EnemyDingo", "Dingo") .SetSprites("Characters/Enemy_Dingo.png", "Backgrounds/Enemy_Dingo_Background.png") .SetStats(4, 2, 2) .WithCardType("Enemy") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.traits = new List () { TStack("Hunter", 1) }; }) ); #endregion #region Kory assets.Add( new CardDataBuilder(this).CreateUnit("EnemyKory", "Kory") .SetSprites("Characters/Enemy_Dingo.png", "Backgrounds/Enemy_Dingo_Background.png") .SetStats(6) .WithCardType("Enemy") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { //SStack("When Sacrificed Apply Spice To Applier", 1) }; }) ); #endregion #endregion #region Items #region Old Hatchet assets.Add( new CardDataBuilder(this).CreateItem("hatchet", "Old Hatchet") .SetSprites("Items/WandererTribe_CardArt_Hatchet.png", "Backgrounds/WandererTribe_ItemBG_Hatchet.png") .SetDamage(3) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Flimsy", 1) }; }) ); #endregion #region Frozen Fire assets.Add( new CardDataBuilder(this).CreateItem("frozenfire", "Frozen Fire") .SetSprites("Items/WandererTribe_CardArt_FrozenFire.png", "Backgrounds/WandererTribe_ItemBG_FrozenFire.png") .SetTraits(TStack("Aimless", 1)) .SetStartWithEffect(SStack("MultiHit", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect StatusEffectSnow snow = TryGet ("Snow"); snow.targetConstraints.AddItem(ScriptableObject.CreateInstance ()); data.attackEffects = new CardData.StatusEffectStacks[] { new CardData.StatusEffectStacks(snow, 2) }; }) ); #endregion #region Rations assets.Add( new CardDataBuilder(this).CreateItem("rations", "Rations") .SetSprites("Items/WandererTribe_CardArt_Rations.png", "Backgrounds/WandererTribe_ItemBG_Rations.png") .SetTraits(TStack("Aimless", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("MultiHit", 3), SStack("Reduce Frenzy On Use", 1) }; StatusEffectInstantIncreaseMaxHealth increaseHealth = TryGet ("Increase Max Health"); data.attackEffects = new CardData.StatusEffectStacks[] { new CardData.StatusEffectStacks(increaseHealth, 1) }; }) ); #endregion #region Playing Cards assets.Add( new CardDataBuilder(this).CreateItem("playingcards", "Playing Cards") .SetSprites("Items/WandererTribe_CardArt_PlayingCards.png", "Backgrounds/WandererTribe_ItemBG_PlayingCards.png") .SetTraits(TStack("Aimless", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("MultiHit", 1) }; StatusEffectInstantIncreaseAttack increaseAttack = TryGet ("Increase Attack"); increaseAttack.targetConstraints.AddItem(ScriptableObject.CreateInstance ()); data.attackEffects = new CardData.StatusEffectStacks[] { new CardData.StatusEffectStacks(increaseAttack, 1) }; }) ); #endregion #region Sewing Kit assets.Add(CardCopy("FallowMask", "sewingkit") .WithTitle("Sewing Kit") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Plush Monster", 1) }; }) ); #endregion #region Whiskey assets.Add(CardCopy("EyeDrops", "Whiskey") .WithTitle("Whiskey") .SetSprites("Items/WandererTribe_CardArt_Whiskey.png", "Backgrounds/WandererTribe_ItemBG_Whiskey.png") .WithValue(50) .SetAttackEffect(SStack("Increase Attack", 5), SStack("Instant Gain Aimless", 1)) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); }) ); #endregion #region Pack O' Smokes assets.Add(CardCopy("PinkberryJuice", "Smokes") .WithTitle("Pack O' Smokes") .SetSprites("Items/WandererTribe_CardArt_Smokes.png", "Backgrounds/WandererTribe_ItemBG_Smokes.png") .WithValue(40) .SetAttackEffect(SStack("Heal", 8)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Reduce Effects To Self", 1) }; }) ); #endregion #region Painkillers assets.Add(new CardDataBuilder(this) .CreateItem("Painkillers", "Painkillers") .SetSprites("Items/WandererTribe_CardArt_Painkillers.png", "Backgrounds/WandererTribe_ItemBG_Painkillers.png") .WithValue(55) .SetAttackEffect(SStack("Block", 2)) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Hand allowed data.canPlayOnHand = true; }) ); #endregion #region Confusing Map assets.Add(new CardDataBuilder(this) .CreateItem("ConfusingMap", "Confusing Map") .SetSprites("Items/WandererTribe_CardArt_ConfusingMap.png", "Backgrounds/WandererTribe_ItemBG_ConfusingMap.png") .WithValue(55) .NeedsTarget(false) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.canPlayOnBoard = false; data.canPlayOnEnemy = false; data.canPlayOnFriendly = false; // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Add Noomlin To Random Card In Hand", 1), SStack("MultiHit", 1) }; }) ); #endregion #region Soul Candy assets.Add(new CardDataBuilder(this) .CreateItem("SoulCandy", "Soul Candy") .SetSprites("Items/WandererTribe_CardArt_SoulCandy.png", "Backgrounds/WandererTribe_ItemBG_SoulCandy.png") .WithValue(45) .SetAttackEffect(SStack("Heal", 2)) .SetTraits(TStack("Noomlin", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Hand allowed data.canPlayOnHand = true; }) ); #endregion #region A Book assets.Add(new CardDataBuilder(this) .CreateItem("Book", "A Book") .SetSprites("Items/WandererTribe_CardArt_ABook.png", "Backgrounds/WandererTribe_ItemBG_ABook.png") .WithValue(40) .NeedsTarget(false) .SetTraits(TStack("Draw", 3)) ); #endregion #region Rage Brew assets.Add(new CardDataBuilder(this) .CreateItem("RageBrew", "Rage Brew") .SetSprites("Items/WandererTribe_CardArt_RageBrew.png", "Backgrounds/WandererTribe_ItemBG_RageBrew.png") .WithValue(50) .SetStats(null, 3) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); data.attackEffects = new CardData.StatusEffectStacks[] { SStack("Double Attack Effect", 1), SStack("Instant Gain Hunter", 1) }; }) ); #endregion #region Merciful Dagger assets.Add(new CardDataBuilder(this) .CreateItem("MercifulDagger", "Merciful Dagger") .SetSprites("Items/WandererTribe_CardArt_MercifulDagger.png", "Backgrounds/WandererTribe_ItemBG_MercifulDagger.png") .WithValue(50) .SetStats(null, 0) .CanPlayOnEnemy(false) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); data.attackEffects = new CardData.StatusEffectStacks[] { SStack("Sacrifice Ally", 1), SStack("Instant Apply Current Health And Attack To Front Ally", 1) }; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Flimsy", 1) }; }) ); #endregion #region Cutlass assets.Add(new CardDataBuilder(this) .CreateItem("Cutlass", "Cutlass") .SetSprites("Items/WandererTribe_CardArt_Cutlass.png", "Backgrounds/WandererTribe_ItemBG_Cutlass.png") .WithValue(45) .SetStats(null, 2) .SetTraits(TStack("Greed", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Hit Equal Gain Gold", 1) }; }) ); #endregion #region Worn Dice assets.Add(new CardDataBuilder(this) .CreateItem("WornDice", "Worn Dice") .SetSprites("Items/WandererTribe_CardArt_WornDice.png", "Backgrounds/WandererTribe_ItemBG_WornDice.png") .WithValue(40) .SetStartWithEffect(SStack("MultiHit", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); data.attackEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Random Damage", 1) }; }) ); #endregion #region Quill assets.Add(new CardDataBuilder(this) .CreateItem("Quill", "Quill") .SetSprites("Items/WandererTribe_CardArt_Quill.png", "Backgrounds/WandererTribe_ItemBG_Quill.png") .WithValue(50) .SetStats(null, 1) .SetAttackEffect(SStack("Null", 3)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); }) ); #endregion #region Foul Soup assets.Add(new CardDataBuilder(this) .CreateItem("FoulSoup", "Foul Soup") .SetSprites("Items/WandererTribe_CardArt_FoulSoup.png", "Backgrounds/WandererTribe_ItemBG_FoulSoup.png") .WithValue(60) .SetTraits(TStack("Aimless", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect StatusEffectHaze haze = TryGet ("Haze"); haze.targetConstraints.AddItem(ScriptableObject.CreateInstance ()); data.attackEffects = new CardData.StatusEffectStacks[] { new CardData.StatusEffectStacks(haze, 1) }; }) ); #endregion #region Hunting Spear assets.Add(new CardDataBuilder(this) .CreateItem("HuntingSpear", "Hunting Spear") .SetSprites("Items/WandererTribe_CardArt_HuntingSpear.png", "Backgrounds/WandererTribe_ItemBG_HuntingSpear.png") .WithValue(40) .SetStats(null, 5) .SetStartWithEffect(SStack("On Kill Apply Attack To Self", 2)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); }) ); #endregion #region Thermal Tracker assets.Add(CardCopy("Leecher", "ThermalTracker") .WithTitle("Thermal Tracker") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .WithValue(50) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { data.traits.Clear(); data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Enemy Innocent Monster", 1) }; }) ); #endregion #region Tom's Pickaxe assets.Add(new CardDataBuilder(this) .CreateItem("Pickaxe", "Tom's Pickaxe") .SetSprites("Items/WandererTribe_CardArt_TomsPickaxe.png", "Backgrounds/WandererTribe_ItemBG_TomsPickaxe.png") .WithValue(40) .SetStats(null, 3) .SetAttackEffect(SStack("Snow", 2)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); }) ); #endregion #region Volatile Vial assets.Add(new CardDataBuilder(this) .CreateItem("VolatileVial", "Volatile Vial") .SetSprites("Items/WandererTribe_CardArt_VolatileVial.png", "Backgrounds/WandererTribe_ItemBG_VolatileVial.png") .WithValue(50) .SetStats(null, 0) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Hex", 1) }; data.traits.Add(TStack("Splash", 1)); }) ); #endregion #region Barbed Wire assets.Add(new CardDataBuilder(this) .CreateItem("BarbedWire", "Barbed Wire") .SetSprites("Items/WandererTribe_CardArt_BarbedWire.png", "Backgrounds/WandererTribe_ItemBG_BarbedWire.png") .WithValue(45) .SetStats(null, 1) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); data.attackEffects = new CardData.StatusEffectStacks[] { SStack("Teeth", 2) }; }) ); #endregion #region Sun-baked Cookies assets.Add(new CardDataBuilder(this) .CreateItem("SunCookies", "Sun-baked Cookies") .SetSprites("Items/WandererTribe_CardArt_SunBakedCookies.png", "Backgrounds/WandererTribe_ItemBG_SunBakedCookies.png") .WithValue(50) .SetAttackEffect(SStack("Reduce Counter", 1)) .SetStartWithEffect(SStack("MultiHit", 1)) .SetTraits(TStack("Combo", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); }) ); #endregion #region Scythe Shard assets.Add(CardCopy("FallowMask", "ScytheShard") .WithTitle("Scythe Shard") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .WithValue(50) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Frozen Corpse", 1) }; }) ); #endregion #region Paid Contract assets.Add(CardCopy("FallowMask", "PaidContract") .WithTitle("Paid Contract") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .WithValue(40) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Random Wanderer Companion", 1) }; }) ); #endregion #region Paperwork assets.Add(new CardDataBuilder(this) .CreateItem("Paperwork", "Paperwork") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .SetStats(null, 1) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Stackable", 1) }; }) ); #endregion #region Good Soup assets.Add(new CardDataBuilder(this) .CreateItem("GoodSoup", "Good Soup") .SetSprites("Items/WandererTribe_CardArt_GoodSoup.png", "Backgrounds/WandererTribe_ItemBG_GoodSoup.png") .WithValue(55) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Hand allowed data.canPlayOnHand = true; // Effect data.attackEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Apply Spice Equal To Health", 1) }; }) ); #endregion #region BOMB assets.Add(new CardDataBuilder(this) .CreateItem("Bomb", "BOMB") .SetSprites("Items/WandererTribe_CardArt_BOMB.png", "Backgrounds/WandererTribe_ItemBG_BOMB.png") .WithValue(50) .SetStats(null, 2) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.attackEffects = new CardData.StatusEffectStacks[] { SStack("On Hit Gain Gold", 1) }; data.traits.Add(TStack("Splash", 1)); }) ); #endregion #region Religious Text assets.Add(new CardDataBuilder(this) .CreateItem("ReligiousText", "Religious Text") .SetSprites("Items/WandererTribe_CardArt_ReligiousText.png", "Backgrounds/WandererTribe_ItemBG_ReligiousText.png") .WithValue(55) .SetStats(null, 0) .SetAttackEffect(SStack("Demonize", 1)) .SetStartWithEffect(SStack("Hit All Enemies", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); }) ); #endregion #region Otherworldly Feather assets.Add(new CardDataBuilder(this) .CreateItem("OtherworldlyFeather", "Otherworldly Feather") .SetSprites("Items/WandererTribe_CardArt_OtherworldFeather.png", "Backgrounds/WandererTribe_ItemBG_OtherworldFeather.png") .WithValue(50) .SetAttackEffect(SStack("Overload", 3)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Boost To Self", 1) }; }) ); #endregion #region Haunted Sword assets.Add(new CardDataBuilder(this) .CreateItem("HauntedSword", "Haunted Sword") .SetSprites("Items/WandererTribe_CardArt_HauntedSword.png", "Backgrounds/WandererTribe_ItemBG_HauntedSword.png") .WithValue(50) .SetStats(null, 0) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Hit Apply Overload Equal To Target Attack", 1) }; }) ); #endregion #region Assorted Peppers assets.Add(new CardDataBuilder(this) .CreateItem("AssortedPeppers", "Assorted Peppers") .SetSprites("Items/WandererTribe_CardArt_AssortedPeppers.png", "Backgrounds/WandererTribe_ItemBG_AssortedPeppers.png") .WithValue(45) .SetAttackEffect(SStack("Spice", 2)) .SetStartWithEffect(SStack("MultiHit", 2)) .SetTraits(TStack("Aimless", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); }) ); #endregion #region Foraging Basket assets.Add(new CardDataBuilder(this) .CreateItem("ForagingBasket", "Foraging Basket") .SetSprites("Items/WandererTribe_CardArt_ForagingBasket.png", "Backgrounds/WandererTribe_CardArt_ForagingBasket.png") .WithValue(50) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.attackEffects = new CardData.StatusEffectStacks[] { SStack("Apply Shroom Equal To Ally Count", 1) }; }) ); #endregion #region Spoiled Rations assets.Add( new CardDataBuilder(this).CreateItem("SpoiledRations", "Spoiled Rations") .SetSprites("Items/WandererTribe_CardArt_SpoiledRations.png", "Backgrounds/WandererTribe_ItemBG_SpoiledRations.png") .WithValue(50) .SetAttackEffect(SStack("Shroom", 2)) .SetTraits(TStack("Aimless", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("MultiHit", 3), SStack("Reduce Frenzy On Use", 1) }; }) ); #endregion #region Tiny Snowman assets.Add( new CardDataBuilder(this).CreateItem("TinySnowman", "Tiny Snowman") .SetSprites("Items/WandererTribe_CardArt_TinySnowman.png", "Backgrounds/WandererTribe_ItemBG_TinySnowman.png") .SetAttackEffect(SStack("Snow", 1)) .SetTraits(TStack("Zoomlin", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Add Tiny Snowman To Hand", 1), SStack("Stackable", 1) }; }) ); #endregion #region Massive Snowflake assets.Add( new CardDataBuilder(this).CreateItem("MassiveSnowflake", "Massive Snowflake") .SetSprites("Items/WandererTribe_CardArt_MassiveSnowflake.png", "Backgrounds/WandererTribe_ItemBG_MassiveSnowflake.png") .WithValue(60) .SetStats(null, 0) .SetAttackEffect(SStack("Snow", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Flimsy", 1), SStack("Hit All Enemies", 1) }; }) ); #endregion #region Bottled Dream assets.Add(CardCopy("FallowMask", "BottledDream") .WithTitle("Bottled Dream") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .WithValue(50) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Nightmare", 1) }; }) ); #endregion #region Tasty Page assets.Add(CardCopy("FallowMask", "TastyPage") .WithTitle("Tasty Page") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .WithValue(50) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Knowledge Gremlin", 1) }; }) ); #endregion #region Cursed Scythe assets.Add(new CardDataBuilder(this) .CreateItem("CursedScythe", "Cursed Scythe") .SetSprites("Items/WandererTribe_CardArt_CursedScythe.png", "Backgrounds/WandererTribe_ItemBG_CursedScythe.png") .SetStats(null, 10) .WithValue(50) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Effect data.attackEffects = new CardData.StatusEffectStacks[] { SStack("Instant Apply Hex To Random Ally", 1) }; data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("On Card Played Boost To Self", 1) }; }) ); #endregion #region Cage assets.Add(CardCopy("FallowMask", "Cage") .WithTitle("Cage") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .WithValue(50) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Savage Beast", 1), SStack("Gain Zoomlin When Redraw Charges", 1) }; }) ); #endregion #region Blood Trail assets.Add(CardCopy("FallowMask", "BloodTrail") .WithTitle("Blood Trail") .SetSprites("Items/WandererTribe_CardArt_SewingKit.png", "Backgrounds/WandererTribe_ItemBG_SewingKit.png") .WithValue(55) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Ravenous Creature", 1) }; }) ); #endregion #region Faint Shadow assets.Add(CardCopy("FallowMask", "FaintShadow") .WithTitle("Faint Shadow") .SetSprites("Items/WandererTribe_CardArt_FaintShadow.png", "Backgrounds/WandererTribe_ItemBG_FaintShadow.png") .WithValue(65) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Effect data.startWithEffects = new CardData.StatusEffectStacks[] { SStack("Summon Other Leaders", 1) }; }) ); #endregion #region Snake Oil assets.Add(new CardDataBuilder(this) .CreateItem("SnakeOil", "Snake Oil") .SetSprites("Items/WandererTribe_CardArt_SnakeOil.png", "Backgrounds/WandererTribe_ItemBG_SnakeOil.png") .WithValue(60) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Hand allowed data.canPlayOnHand = true; // Effect data.attackEffects = new CardData.StatusEffectStacks[] { SStack("MultiHit", 1), SStack("Reduce Max Health", 3) }; }) ); #endregion #region Soothing Tea assets.Add(new CardDataBuilder(this) .CreateItem("SoothingTea", "Soothing Tea") .SetSprites("Items/WandererTribe_CardArt_SoothingTea.png", "Backgrounds/WandererTribe_ItemBG_SoothingTea.png") .WithValue(50) .SetTraits(TStack("Consume", 1)) .SubscribeToAfterAllBuildEvent(delegate (CardData data) { // Prevent targeting Intangible data.targetConstraints = data.targetConstraints.AddToArray(ScriptableObject.CreateInstance ()); // Hand allowed data.canPlayOnHand = true; data.attackEffects = new CardData.StatusEffectStacks[] { SStack("Increase Max Health", 8) }; }) ); #endregion #endregion #region Charms #region Predator Charm assets.Add(new CardUpgradeDataBuilder(this) .Create("CardUpgradeHunter") .WithType(CardUpgradeData.Type.Charm) .WithImage("Sprites/hex_icon.png") .WithTitle("Predator Charm") .WithText($"Gain ") .WithTier(1) .SubscribeToAfterAllBuildEvent(delegate (CardUpgradeData data) { // Effect data.giveTraits = new CardData.TraitStacks[] { TStack("Hunter", 1) }; // Constraints data.targetConstraints = new TargetConstraint[] { TryGetConstraint("Does Not Have Aimless"), TryGetConstraint("Does Not Have Barrage"), TryGetConstraint("Does Not Have Longshot"), TryGetConstraint("Is Unit"), TryGetConstraint("Does Attack"), TryGetConstraint("Does Not Have Hit All Enemies") }; }) ); #endregion #region Grotesque Charm assets.Add(new CardUpgradeDataBuilder(this) .Create("CardUpgradeScavenger") .WithType(CardUpgradeData.Type.Charm) .WithImage("Sprites/hex_icon.png") .WithTitle("Grotesque Charm") .WithText($"Gain <1>") .WithTier(3) .SubscribeToAfterAllBuildEvent(delegate (CardUpgradeData data) { // Effect data.giveTraits = new CardData.TraitStacks[] { TStack("Scavenger", 1) }; // Constraints data.targetConstraints = new TargetConstraint[] { TryGetConstraint("Is Unit"), TryGetConstraint("Has Health"), TryGetConstraint("Does Damage") }; }) ); #endregion #region Bewitching Charm assets.Add(new CardUpgradeDataBuilder(this) .Create("CardUpgradeHex") .WithType(CardUpgradeData.Type.Charm) .WithImage("Sprites/hex_icon.png") .WithTitle("Bewitching Charm") .WithText($"Apply <1> ") .WithTier(2) .SubscribeToAfterAllBuildEvent(delegate (CardUpgradeData data) { // Effect data.effects = new CardData.StatusEffectStacks[] { SStack("Hex", 1) }; // Constraints data.targetConstraints = new TargetConstraint[] { TryGetConstraint("Does Trigger"), TryGetConstraint("Does Not Play On Slot"), TryGetConstraint("Plays On Board") }; }) ); #endregion #region ??? Charm assets.Add(new CardUpgradeDataBuilder(this) .Create("CardUpgradeSplash") .WithType(CardUpgradeData.Type.Charm) .WithImage("Sprites/hex_icon.png") .WithTitle("[Unnamed] Charm") .WithText($"Gain ") .WithTier(3) .SubscribeToAfterAllBuildEvent(delegate (CardUpgradeData data) { // Effect data.giveTraits = new CardData.TraitStacks[] { TStack("Splash", 1) }; // Constraints data.targetConstraints = new TargetConstraint[] { TryGetConstraint("Does Not Have Aimless"), TryGetConstraint("Does Not Have Barrage"), TryGetConstraint("Does Not Have Longshot"), TryGetConstraint("Is Unit Or Needs Target"), TryGetConstraint("Does Attack"), TryGetConstraint("Plays On Board"), TryGetConstraint("Does Not Play On Slot"), TryGetConstraint("Does Not Have Instant Summon Copy"), TryGetConstraint("Does Not Have Instant Summon Copy Of Enemy"), TryGetConstraint("Does Not Have Sacrifice Ally"), TryGetConstraint("Does Not Have Hit All Enemies") }; }) ); #endregion #region ??? Charm assets.Add(new CardUpgradeDataBuilder(this) .Create("CardUpgradeAdaptive") .WithType(CardUpgradeData.Type.Charm) .WithImage("Sprites/hex_icon.png") .WithTitle("[Unnamed] Charm") .WithText($"Gain ") .WithTier(3) .SubscribeToAfterAllBuildEvent(delegate (CardUpgradeData data) { // Effect data.effects = new CardData.StatusEffectStacks[] { SStack("Adaptive", 1) }; // Constraints data.targetConstraints = new TargetConstraint[] { TryGetConstraint("Does Not Have Aimless"), TryGetConstraint("Does Trigger"), TryGetConstraint("Is Unit"), TryGetConstraint("Does Attack") }; }) ); #endregion #endregion #region Keywords #region Hex assets.Add( new KeywordDataBuilder(this) .Create("hex") .WithTitle("Hex") .WithTitleColour(new Color(0.85f, 0.44f, 0.85f)) .WithShowName(false) .WithDescription("Apply a random debuff|Debuffs are Bom, Demonize, Frost, Haze, Ink, Overburn, Shroom, and Snow") .WithNoteColour(new Color(0.85f, 0.44f, 0.85f)) .WithBodyColour(new Color(1f, 1f, 1f)) .WithIconName("hexicon") .WithCanStack(true) ); #endregion #region Splash assets.Add( new KeywordDataBuilder(this) .Create("splash") .WithTitle("Splash") .WithTitleColour(new Color(1f, 0.79f, 0.34f)) .WithShowName(true) .WithDescription("Hits adjacent targets") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(false) ); #endregion #region Flimsy assets.Add( new KeywordDataBuilder(this) .Create("flimsy") .WithTitle("Flimsy") .WithTitleColour(new Color(0.5f, 0.5f, 0.5f)) .WithShowName(true) .WithDescription("50% chance to on use") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(false) ); #endregion #region Mimic assets.Add( new KeywordDataBuilder(this) .Create("mimic") .WithTitle("Mimic") .WithTitleColour(new Color(1f, 0.79f, 0.34f)) .WithShowName(true) .WithDescription("Copy the effects of the enemy hit|Effects last until new effects are copied") .WithBodyColour(new Color(1f, 1f, 1f)) .WithNoteColour(new Color(1f, 0.79f, 0.34f)) .WithCanStack(false) ); #endregion #region Adaptive assets.Add( new KeywordDataBuilder(this) .Create("adaptive") .WithTitle("Adaptive") .WithTitleColour(new Color(1f, 0.79f, 0.34f)) .WithShowName(true) .WithDescription($"Gain when placed in front\nGain when placed in middle\nGain when placed in back") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(false) ); #endregion #region Scavenger assets.Add( new KeywordDataBuilder(this) .Create("scavenger") .WithTitle("Scavenger") .WithTitleColour(new Color(0.6f, 0.1f, 0.1f)) .WithShowName(true) .WithDescription("When an ally dies, gain and ") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(true) ); #endregion #region Lion assets.Add( new KeywordDataBuilder(this) .Create("lion") .WithTitle("Lion") .WithTitleColour(new Color(1f, 0.79f, 0.34f)) .WithShowName(true) .WithDescription($"Lions are allies that include , , and ") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(false) ); #endregion #region Hunter assets.Add( new KeywordDataBuilder(this) .Create("hunter") .WithTitle("Hunter") .WithTitleColour(new Color(1f, 0.79f, 0.34f)) .WithShowName(true) .WithDescription("Always hits the lowest enemy in the row") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(false) ); #endregion #region Execute assets.Add( new KeywordDataBuilder(this) .Create("execute") .WithTitle("Execute") .WithTitleColour(new Color(0.75f, 0f, 0f)) .WithShowName(true) .WithDescription("Kills the target|Does not work against Clunkers") .WithBodyColour(new Color(1f, 1f, 1f)) .WithNoteColour(new Color(0.75f, 0f, 0f)) .WithCanStack(false) ); #endregion #region Secretary assets.Add( new KeywordDataBuilder(this) .Create("secretary") .WithTitle("Secretary") .WithTitleColour(new Color(1f, 0.79f, 0.34f)) .WithShowName(true) .WithDescription($"Add to your hand") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(true) ); #endregion #region Stackable assets.Add( new KeywordDataBuilder(this) .Create("stackable") .WithTitle("Stackable") .WithTitleColour(new Color(1f, 0.79f, 0.34f)) .WithShowName(true) .WithDescription("When another copy of this card is created while it is in your hand, combine the card and the copy together") .WithBodyColour(new Color(1f, 1f, 1f)) ); #endregion #region Bloodlust assets.Add( new KeywordDataBuilder(this) .Create("bloodlust") .WithTitle("Bloodlust") .WithTitleColour(new Color(1f, 0f, 0f)) .WithShowName(true) .WithDescription("Gain on kill\nLose after attack ends") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(false) ); #endregion #region Eclipse assets.Add( new KeywordDataBuilder(this) .Create("eclipse") .WithTitle("Eclipse") .WithTitleColour(new Color(0.5f, 0.5f, 0.5f)) .WithShowName(true) .WithDescription("Counts up the target's and counts down by the same amount") .WithCanStack(true) ); #endregion #region Intangible assets.Add( new KeywordDataBuilder(this) .Create("intangible") .WithTitle("Intangible") .WithDescription("Cannot be hit") .WithTitleColour(new Color(0.5f, 1f, 0.66f)) .WithShowName(true) ); #endregion #region Nocturnal assets.Add( new KeywordDataBuilder(this) .Create("nocturnal") .WithTitle("Nocturnal") .WithDescription("Unaffected by count down and trigger effects") .WithTitleColour(new Color(0.5f, 0.5f, 0.5f)) .WithShowName(true) ); #endregion #region Consumed assets.Add( new KeywordDataBuilder(this) .Create("consumed") .WithTitle("Consumed") .WithDescription("The condition when a card with is played") .WithShowName(true) ); #endregion #region Lions assets.Add( new KeywordDataBuilder(this) .Create("lions") .WithTitle("Lions") .WithTitleColour(new Color(1f, 0.79f, 0.34f)) .WithShowName(true) .WithDescription($"Lions are allies that include , , and ") .WithBodyColour(new Color(1f, 1f, 1f)) .WithCanStack(false) ); #endregion #region Consumable assets.Add( new KeywordDataBuilder(this) .Create("consumable") .WithTitle("Consumable") .WithDescription(" when placed") .WithShowName(true) ); #endregion #region Bless assets.Add( new KeywordDataBuilder(this) .Create("bless") .WithTitle("Bless") .WithTitleColour(new Color(1f, 1f, 0.7f)) .WithShowName(false) .WithDescription("Apply a random buff|Buffs are Block, Shell, Spice, and Teeth") .WithNoteColour(new Color(1f, 1f, 0.7f)) .WithBodyColour(new Color(1f, 1f, 1f)) .WithIconName("hexicon") .WithCanStack(true) ); #endregion #endregion #region Traits #region Hunter Trait assets.Add(new TraitDataBuilder(this) .Create("Hunter") .SubscribeToAfterAllBuildEvent(delegate (TraitData data) { data.keyword = TryGet ("hunter"); data.overrides = new TraitData[] { TryGet ("Aimless"), TryGet ("Longshot"), TryGet ("Barrage"), TryGet ("Splash") }; data.effects = new StatusEffectData[] { TryGet ("Hit Lowest Health Enemy In Row") }; }) ); #endregion #region Splash Trait assets.Add(new TraitDataBuilder(this) .Create("Splash") .SubscribeToAfterAllBuildEvent(delegate (TraitData data) { data.keyword = TryGet ("splash"); data.overrides = new TraitData[] { TryGet ("Aimless"), TryGet ("Longshot"), TryGet ("Barrage"), TryGet ("Hunter") }; data.effects = new StatusEffectData[] { TryGet ("Hit Adjacent Enemies")}; }) ); #endregion #region Scavenger Trait assets.Add(new TraitDataBuilder(this) .Create("Scavenger") .SubscribeToAfterAllBuildEvent(delegate (TraitData data) { data.keyword = TryGet ("scavenger"); data.effects = new StatusEffectData[] { TryGet ("When Ally Is Killed Gain Attack And Health") }; }) ); #endregion #endregion #region Effects #region Keyword Effects #region Hex Effect assets.Add(StatusCopy("Snow","Hex") .Create ("Hex") .WithCanBeBoosted(true) .WithText("Apply <{a}> ") .WithType("") .FreeModify (delegate (StatusEffectApplyRandom data) { string[] effects = { "Weakness", "Demonize", "Frost", "Haze", "Null", "Overload", "Shroom", "Snow" }; data.effectsToApply = effects; ((StatusEffectApplyX)data).applyToFlags = StatusEffectApplyX.ApplyToFlags.Target; }) ); #endregion #region Flimsy Effect assets.Add(StatusCopy("Destroy After Use", "Flimsy") .Create ("Flimsy") .WithCanBeBoosted(false) .WithText($" ") .WithType("") ); #endregion #region Mimic Effect assets.Add(new StatusEffectDataBuilder(this) .Create ("On Card Played Copy Effects") .WithCanBeBoosted(false) .WithText($" ") .SubscribeToAfterAllBuildEvent(delegate (StatusEffectData data) { ((StatusEffectApplyX)data).queue = true; ((StatusEffectApplyX)data).applyToFlags = StatusEffectApplyX.ApplyToFlags.Target; ((StatusEffectApplyX)data).effectToApply = TryGet ("Copy Effects Repeatable"); }) ); #endregion #region Mimic's Indirect Effect assets.Add( new StatusEffectDataBuilder(this) .Create ("Copy Effects Repeatable") .WithCanBeBoosted(true) ); #endregion #region Adaptive Effect assets.Add(new StatusEffectDataBuilder(this) .Create ("Adaptive") .WithCanBeBoosted(false) .WithText($" ") .SubscribeToAfterAllBuildEvent(delegate (StatusEffectData data) { ((StatusEffectApplyXOnMove)data).traitsToApply = new TraitData[] { TryGet ("Smackback"), TryGet ("Hunter"), TryGet ("Longshot") }; ((StatusEffectApplyX)data).applyToFlags = StatusEffectApplyX.ApplyToFlags.Self; }) ); #endregion #region Scavenger Effect assets.Add(new StatusEffectDataBuilder(this) .Create ("When Ally Is Killed Gain Attack And Health") .WithCanBeBoosted(true) .WithStackable(true) .SubscribeToAfterAllBuildEvent(delegate (StatusEffectData data) { ((StatusEffectApplyX)data).applyToFlags = StatusEffectApplyX.ApplyToFlags.Self; ((StatusEffectApplyX)data).effectToApply = TryGet ("Increase Attack & Health"); }) ); #endregion #region Execute Effect assets.Add(new StatusEffectDataBuilder(this) .Create ("Instant Kill") .WithText($" ") .SubscribeToAfterAllBuildEvent(delegate (StatusEffectData data) { TargetConstraintHasStatus notScrap = ScriptableObject.CreateInstance (); notScrap.status = TryGet ("Scrap"); notScrap.not = true; ((StatusEffectInstantExecute)data).killConstraints = new TargetConstraint[] { notScrap }; }) ); #endregion #region Hunter Effect assets.Add(new StatusEffectDataBuilder(this) .Create ("Hit Lowest Health Enemy In Row") .WithCanBeBoosted(false) .SubscribeToAfterAllBuildEvent(delegate (StatusEffectData data) { ((StatusEffectChangeTargetMode)data).targetMode = ScriptableObject.CreateInstance (); }) ); #endregion #region Splash Effect assets.Add(new StatusEffectDataBuilder(this) .Create ("Hit Adjacent Enemies") .WithCanBeBoosted(false) .SubscribeToAfterAllBuildEvent(delegate (StatusEffectData data) { ((StatusEffectChangeTargetMode)data).targetMode = ScriptableObject.CreateInstance